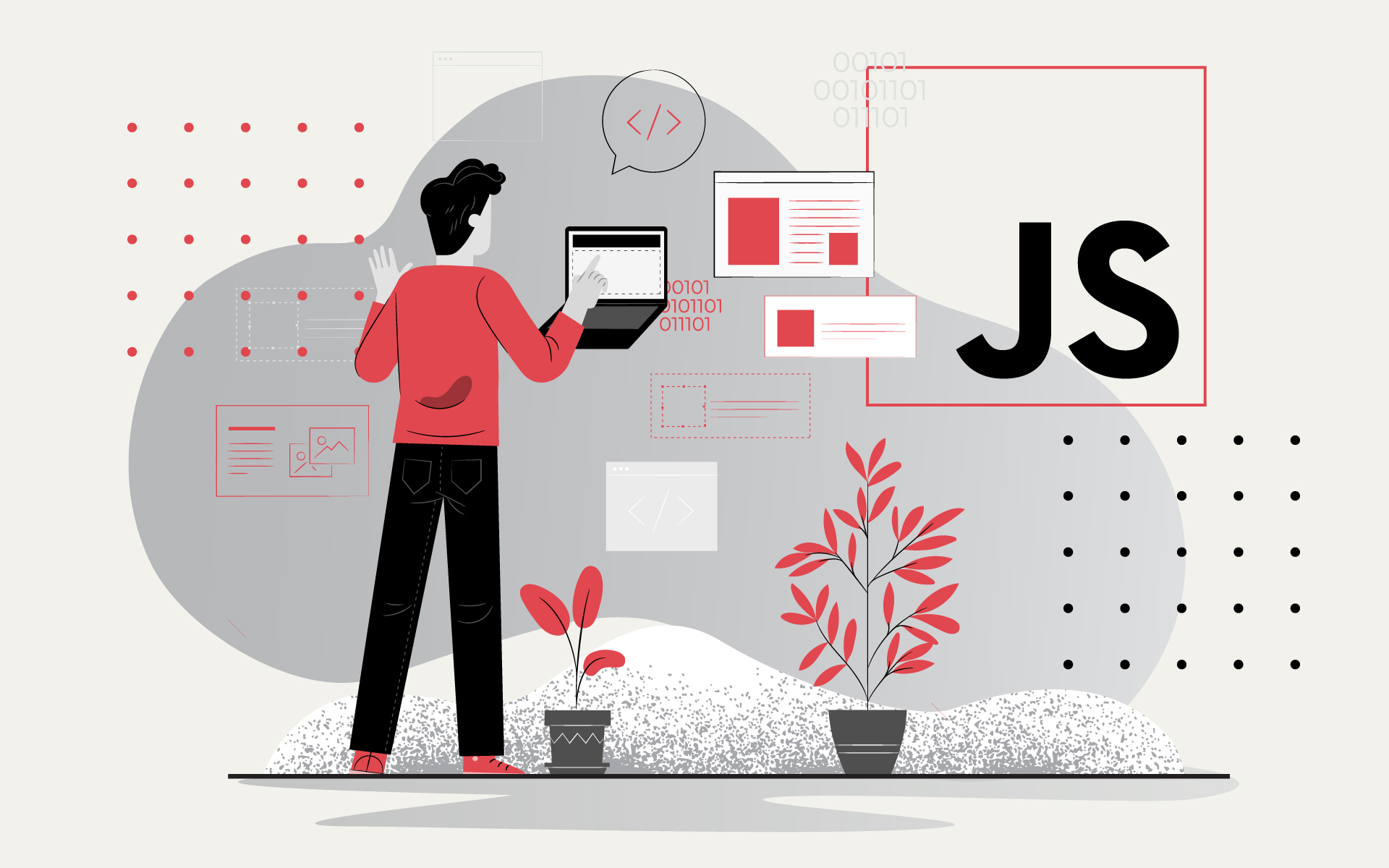
For eight years in a row, JavaScript has been ranked the most popular programming language in the StackOverflow survey. In 2020, it was used by 67,7% of the respondents.
What are the reasons for its success?
In this article, we will explain why JavaScript is so popular and tell you everything you need to know to use it for your own project.
What is JavaScript, and where is it used?
JavaScript is an interpreted scripting language that is mainly used on the web.
Historically, there have been three programming languages that you can render in a web browser:
- HTML, which is responsible for the structure of the web page.
- CSS, which is responsible for how the web page looks.
- JavaScript, which is responsible for the dynamic interactions between page elements.
Out of these, JavaScript is responsible for interactive behavior. For example, you can use JavaScript to create animations, carousels, and other awesome stuff. You can even use it to make apps that work right in the browser!
Can you use JavaScript anywhere else?
Nowadays, JavaScript can also be used as a server-side language with frameworks like Node.js and Deno. This means that you can build your whole website in JavaScript, both the server and the parts that are accessible to the users.
You can also create mobile apps with JavaScript. For example, React Native, a framework for developing native apps for Android and iOS, uses JavaScript.
Advantages and disadvantages
Like all programming languages, JS has certain advantages and disadvantages in contrast to other programming languages.
Here, I will assume that we have already decided to create a web solution. In that case, we need to understand the benefits of JavaScript as a client-side language in contrast to other languages that compile to JavaScript, and the benefits of JavaScript as a server-side language.
The main benefits of JavaScript are:
- Dynamic typing. This is very beneficial for creating prototypes quickly, but static typing could be more useful in large scale projects due to the type safety and transparency it provides.
- Accessibility. JavaScript has been around for a long time and has many tutorials, support tools, and other community benefits. In addition, dynamic typing makes it much easier to start building projects as fast as possible.
- Popularity. Since JavaScript is so popular, it is much easier to find the necessary tools and libraries for your project, which boosts developer productivity. Nobody wants to spend time writing extra functionality if they can escape that.
JavaScript also has a few disadvantages.
- Performance issues. JavaScript is a terrible choice for performance-critical tasks where you would want to use a low-level language like C. Fortunately, most of the web apps and pages do not fall into this category.
- JavaScript is not type-safe. A lot of bugs that JS developers run into can be solved by a reliable type system. Fortunately, there are a lot of additional tools like linters that help developers avoid them, and adding static types is as easy as moving to TypeScript, a statically-typed version of JS.
A brief history of JavaScript
Back in 1995, in the midst of browser wars between Netscape Navigator and Microsoft Internet Explorer, a programmer called Brendan Eich came up with an idea of a new scripting language. What is more surprising, he built it in just ten days! First, it was called Mocha, then LiveScript, and finally–JavaScript.
While the name suggests a connection between Java and JavaScript, there’s actually not a lot of similarity between the languages. Back then, Java was very popular, and Netscape made a licensing deal with Sun to ride the hype train. In hindsight, this move worked out quite well.
After some time, the language was submitted to standardization for ECMA International. It’s essential to have standards for a language like JavaScript since different competing browsers can run different engines for interpreting JavaScript. Therefore, all these engines need a common language.
The specification for JavaScript is called ECMAScript (after the standard issuer) or ES in short. That’s why we refer to different versions of JavaScript as ES6, ES10, or other.
Since then, JavaScript has grown together with the web, and at the moment of writing, several resources list it as one of the top programming languages in the world.
JavaScript frameworks
Because of the sheer amount of people building and working on JavaScript, its ecosystem support is astonishing. There are many frameworks out there that make it easier to create web pages and apps easily and quickly.
We’ll list a couple of them:
Angular
Angular is a web app framework led by Angular Team at Google. It is written in TypeScript, a programming language that’s very similar to JavaScript but includes static typing.
As its strengths, Angular lists speed, performance, and well-developed tooling. What is more, Angular has a ton of built-in features–whatever you need for your daily web development, Angular is likely to have it.
In addition, you can use Angular to build web and native mobile and desktop apps.
React
React is a web app framework whose development is led by Facebook. In contrast to Angular, it is much more minimalistic. Therefore, if you need some additional stuff that the framework doesn’t include, you frequently have to search for community-made libraries.
Like Angular, it enables you to make mobile apps with React Native.
Vue.js
Vue.js is a JavaScript framework for building web UIs. It’s very approachable to users who already have been developing with HTML, CSS, and JavaScript. It’s also very versatile, enabling you to use it either as a library or a complete framework.
You can think of Vue as being somewhere in the middle of React and Angular in the number of provided features. In contrast to both of them, Vue was not made by a large company like Google or Facebook. Instead, it is developed and maintained purely by the open-source community.
Node.js
These last two frameworks enable you to run JavaScript outside of the web browser.
Node.js is the more famous one and is a solid choice for any backend JS project. It is based on V8, an open-source JavaScript engine that is used in Chromium browsers like Chrome, Edge, and Opera.
Deno
Deno, in contrast, is much newer and less battle-tested. But according to the creator of Deno, Ryan Dahl (who is also the creator of Node.js), it improves security, TypeScript support, and other Node’s features.
Like Node, it also uses V8. It’s built in Rust.
JavaScript alternatives
While many developers are perfectly fine with writing vanilla JavaScript, there are some languages out there that provide a different kind of programming experience.
Frontend
On the frontend, you can use multiple other alternatives that compile to JavaScript. Since JavaScript is the language of the browser, all of these ultimately compile to JavaScript.
This enables developers to interoperate with JavaScript codebases while keeping the benefits of the language.
Here are the most popular ones:
- TypeScript. Made by Microsoft, TypeScript is not vastly different from JavaScript. It is JavaScript with static typing. Static types enable developers to write more complex code, orient in their code better, and escape some errors and bugs while compiling the program.
- Dart. Dart is a client-optimized programming language for making apps. It was created at Google as an alternative to JavaScript.
- Elm. A little bit different than JavaScript, Elm is a declarative programming language for browser-based GUIs. Elm compiles to HTML, CSS, and JavaScript and is quite functional in nature, which leads it to be frequently compared with languages like Haskell.
- Reason. Reason is a syntax extension for OCaml, made by the same person that came up with React at Facebook. It’s quite similar to other JavaScript alternatives–it adds static typing and feels more functional–but this time with an OCaml feel.
There’s also WebAssembly, but we will delve into the exact details of it below.
Backend
Any other server-side programming language like Java, Python, or Ruby can serve as an alternative for JS on the backend. JS shows similar performance and has the benefit of having the same language on the client and server sides.
JavaScript vs. WASM
A particular innovation in the web browser space is WebAssembly (or WASM in short).
WebAssembly is a portable compilation target that can enable one to compile any programming language to code that runs in the browser. In other words, it lets you write web apps in any language.
In contrast to JavaScript, it is a low-level binary language.
This has serious implications for the future. For example, WebAssembly lets us create apps for the web that would be impossible to do in JavaScript due to performance reasons. While JavaScript's performance is somewhat unreliable, WebAssembly is heavily optimized and can be used to run things like games, graphic editors, and other apps straight in the browser.
But does it mean it is going to be a serious contender to JavaScript?
Most likely, they will exist in a symbiotic relationship, with WebAssembly being used for truly ambitious projects and JavaScript being used for regular web pages and apps.
There are many benefits to JavaScript, such as simplicity and practicality, that make it hard to overthrow it as the main programming language of the web. Therefore, it is still a fast and convenient choice for most programming tasks.
Future of JavaScript
Overall, the future of JavaScript looks bright. With one of the largest ecosystems in the world, it is continuously improved through new tools and frameworks. Since it is basically the only programming language that works on the web, it is also clear that it is not going anywhere.
There are trends towards JavaScript becoming more and more a compile target, though. But, while there are several ambitious projects and alternatives to JavaScript underway, it is not yet clear whether they will be practical enough for broad adoption. In the meantime, JavaScript serves as an excellent common tongue for web developers all around the world.